Table of Contents
Routes in Nuxt js, a powerful framework based on Vue.js, simplifies the process of building server-side rendered (SSR) applications. One of its standout features is the ability to create dynamic routes effortlessly, making it ideal for projects that require flexible and scalable routing solutions. In this guide, we’ll explore how you can leverage Nuxt js to create dynamic routes that adapt to various data sources and user interactions.
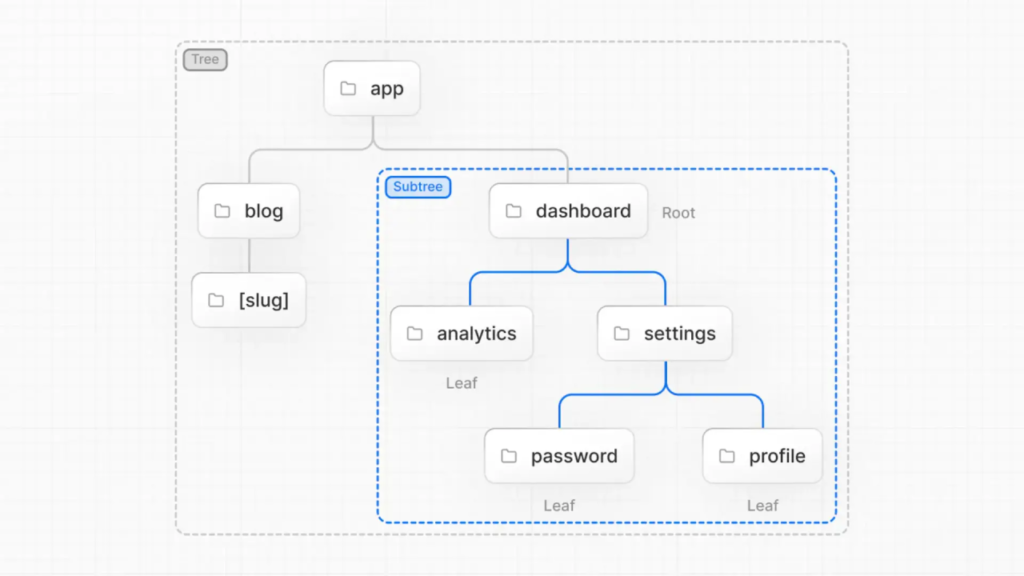
Understanding Dynamic Routes in Nuxt js
Dynamic routes in Nuxt js allow developers to define routes that depend on parameters or variables. Unlike static routes, which are predefined, dynamic routes are generated based on data fetched from an API, a database, or other sources. This flexibility is crucial for applications that handle diverse content or user-specific information.
Benefits of Using Dynamic Routes
Dynamic routes offer several advantages:
1. Flexibility
Dynamic Adaptation:
Dynamic route generation allows your application to respond to real-time data and user interactions, ensuring that routes and content are always up-to-date. This flexibility is crucial in applications where content is frequently changing, such as news websites, e-commerce platforms, or social media apps. For instance, in an e-commerce site, if a new product is added, the application can automatically create a new route for that product without requiring manual intervention. This adaptability ensures that users always have access to the latest content, improving the overall user experience.
2. Scalability
Efficient Management of Large Datasets:
As your application grows, managing large amounts of data or content can become challenging. Dynamically generating routes allows the application to handle this complexity by creating routes on demand. This approach reduces the load on the server and database by only fetching and rendering the necessary data when requested by the user. For example, in a blog platform with thousands of articles, generating routes dynamically ensures that only the requested articles are loaded, rather than preloading all possible routes. This not only improves performance but also makes it easier to scale the application as more content is added.
3. SEO Optimization
Search Engine-Friendly URLs:
Dynamic routes can significantly enhance your application’s SEO by creating URLs that are descriptive and relevant to the content. Search engines prefer URLs that include keywords related to the content they represent, making them more likely to rank higher in search results. For example, instead of a generic URL like /product/12345
, a dynamic route can create a more descriptive URL like /products/smartphone-xyz-model
, which is more likely to be indexed and ranked by search engines. Additionally, dynamic routes can facilitate the creation of sitemaps, further improving the site’s visibility to search engines.
Implementing Dynamic Routes in Nuxt js
To implement dynamic routes in Nuxt js, follow these steps:
Step 1: Define Dynamic Pages
Begin by creating a directory structure within your Nuxt js project to organize dynamic pages. Use the underscore _ prefix for dynamic parameters in your page filenames. For example, a dynamic page for individual blog posts could be named _slug. vue, where slug is a parameter.
Step 2: Fetch Data Dynamically
Use Nuxt js async data fetching methods such as asyncData or fetch in your dynamic page components to retrieve data based on dynamic parameters. This data can come from APIs, databases, or local sources.
Step 3: Generate Dynamic Routes
Nuxt js provides the generate property in nuxt.config.js to dynamically generate routes based on your data. Define a generate function that fetches data and returns an array of dynamic routes.
javascript
Copy code
// nuxt.config.js
export default {
generate: {
routes: async () => {
// Fetch dynamic data (e.g., from an API)
const dynamicData = await fetchDynamicData();
// Generate routes based on fetched data
return dynamicData.map(item => `/dynamic/${item.id}`);
}
}
}
Step 4: Navigate Between Dynamic Routes
Use Nuxt js routing methods such as <nuxt-link> or this.$router.push() to navigate between dynamic routes within your application. These methods handle route transitions seamlessly, enhancing user experience.
Advanced Techniques for Dynamic Routes
Handling Nested Dynamic Routes
In complex applications, you may encounter scenarios where routes have nested dynamic segments. Nuxt js supports nested routes by defining nested folder structures and dynamic parameters in page filenames. For instance, /users/_userId/posts/_postId.vue represents a nested dynamic route structure for user-specific posts.
Using Middleware for Dynamic Data
Middleware in Nuxt js allows you to execute code before rendering a page or a group of pages. Utilize middleware to fetch dynamic data required for dynamic routes, ensuring data is available before rendering the corresponding pages.
javascript
Copy code
// middleware/fetchUserData.js
export default async function ({ params, store }) {
const userData = await fetchUserData(params.userId);
store.commit(‘setUserData’, userData);
}
Generating Dynamic Routes with Parameters
Nuxt js enables the generation of dynamic routes with parameters by defining routes dynamically based on fetched data or predefined conditions. Parameters in dynamic routes allow for customization and personalization of content based on user interactions or external data sources.
Conclusion
Creating dynamic routes in Nuxt js empowers developers to build flexible, scalable applications that adapt to changing data and user interactions. By leveraging Nuxt js’ powerful routing capabilities and async data fetching methods, you can create dynamic routes that enhance SEO, improve scalability, and deliver a seamless user experience.
What are dynamic routes in Nuxt js?
Dynamic routes in Nuxt js are routes that are generated based on dynamic data, such as a user’s ID or a product category, allowing the application to serve different content on different URLs without predefining every possible route.
How do I create a dynamic route in Nuxt.js?
To create a dynamic route in Nuxt.js, you need to define a file in the pages
directory with a filename wrapped in square brackets, such as [id].vue
. This tells Nuxt.js that this file is a dynamic route, and the value of id
can change based on the URL.
Can I pass multiple dynamic parameters in a Nuxt js route?
Yes, you can pass multiple dynamic parameters by creating nested dynamic routes. For example, if you need category
and id
, you can structure your files as /pages/[category]/[id].vue
.